One way may be to create a simple mesh around the image and with some clever placement of the UVs, you could make a shader that appears to gradually fade in the line.
So the two vertices in the top-right corner would have the coordinates U coordinate 0.0, bottm-right 0.25, bottom-left 0.5, top-left 0.75, and finally circle back to top-right with 1.0. The V coordinate can stay at 0.0 for all vertices.
Then in a shader, you can the create a 0 to 1 gradient by lerping the saturated U value (so it stays between 0 and 1). By offsetting (adding/subtracting) the result, you can sort of fill-in the shape. If you want a sharp edge, you can use a cutout shader which will clip certain pixels below a specified opacity threshold.
Here's an example surface shader:
Shader "UV Progress"
{
Properties
{
_Color("Color", Color) = (1,1,1,1)
_Progress("Progress", Range( 0 , 1)) = 0
[HideInInspector] _Cutoff( "Mask Clip Value", Float ) = 0.5
[HideInInspector] _texcoord( "", 2D ) = "white" {}
}
SubShader
{
Tags
{
"RenderType" = "TransparentCutout"
"Queue" = "AlphaTest+0"
}
Cull Back
CGPROGRAM
#pragma target 3.0
#pragma surface surf Unlit
struct Input
{
float2 uv_texcoord;
};
float4 _Color;
float _Progress;
float _Cutoff = 0.5;
inline half4 LightingUnlit(SurfaceOutput s, half3 lightDir, half atten)
{
return half4(0, 0, 0, s.Alpha);
}
void surf(Input i, inout SurfaceOutput o)
{
o.Emission = _Color.rgb;
o.Alpha = saturate(i.uv_texcoord.x - 0.5 + _Progress);
clip(o.Alpha - _Cutoff);
}
ENDCG
}
}
Demo of the shader on a simple quad while adjusting the _Progress
property of the shader:
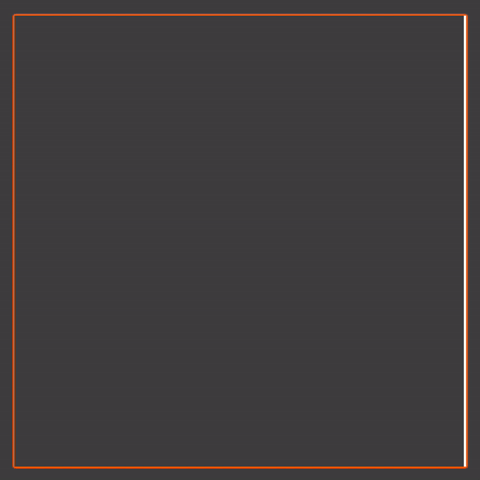