(float)rand.Next(0,1000) / 10
Picks a random number between 0
and 1000
, the divides by 10
. This gives you a random number between 0 and 100. If that random number is less than or equal to your chance (<= .2
), then the statement is true and whatever is inside the if statement is executed.
If you want to get a .2% chance, that means that for every .2% of the times you run this code it will be true. So how do we do that? Pick a random number between 0 and 100, if it's less than .2 it's true.
Throw a random dart at this image:
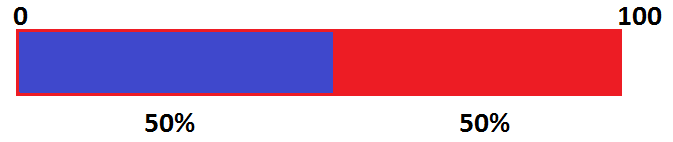
If the dart were truly random, you'd expect it to hit blue 50% of the time and red 50% of the time. So we want something to happen 50% of the time, this is what we would use. So what if we wanted it to happen .2% of the time? I means our image needs to look at bit less equal.
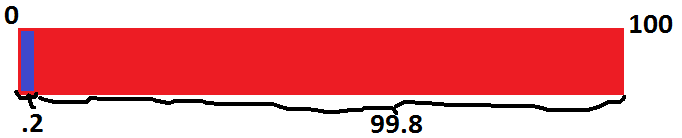
Throw that random dart at this image and you'll hit blue .2% of the time.
EDIT to address your comment
The thing about random numbers is that they don't care about the number that was chosen before them. If you were to flip a fair coin, you have a 50% chance of it landing on heads and a 50% chance of it landing on tails. So should would you be surprised if you flipped it twice in a row and it came up heads both times? No, I don't think you would. How about if you flipped it 6 times, would you be surprised if if came out 4 tails and 2 heads? No that is totally possible. How about 6 heads in a row? Well that is certainly less expected, but it's not impossible. What about 100 flips and they're all heads? Now that seems totally impossible. But it's not, it's just very, very unlikely. Here's the thing though. If you're on flip 99 and they've all been heads up until that point, there's still only a 50% chance that the next flip will be heads too. The next flip has nothing to do with any of the previous flips or any of the flips to come after. Just because you've had 99 heads in a row doesn't make it any more likely that the next flip will be tails (see Gambler's fallacy). However as the sample size grows the number of heads and number of tails will start to equalize. See this simulated coin toss distribition:
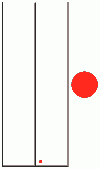
As you can see, there are times where if you stopped right then, things would look very unbalanced. But as the sample size continues to grow, it does start to equalize towards 50/50. You can also see that I got lucky and this animated gif matches the color scheme I picked :).
Probability belongs to statistics. Statistics is one thing that people are typically not good at (if you need proof of that, just look at all the lottery players). It is however very beneficial to study statistics. It'll help you in programming and life in general.
Basically what you should take away from this is:
- Random numbers are not affected by any number that appeared before or after them.
- With small sample sizes, the distribution of probability might look wrong, so don't worry.
- The lottery is a tax for people who don't know statistics
- Random numbers do not guarantee their distribution
- Know statistics well and you will be a superhero