For circle p2
and p3
to be close enough to eachother to touch, we know that the distance between them must be 2 * rs
(where rs
is the radius of the small circles).
If we then construct a triangle from the points p1
, p2
and p3
, we can divide that triagle in the middle and know that the short side of the triangle is equal to rs
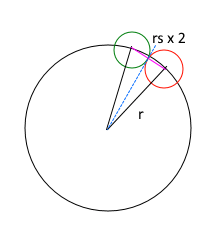
Using the sine rule we can then figure out the angle at the center of the big circle as we have a triangle where we know two of the sides (r
and rs
) and one angle (the 90 degree angle we get from splitting the triangle in half).
This angle times two is the angle between p2
and p3
angle = 2.0 * asin(rs / r)
Since we can get the angle of a Vector2
in libGDX it's then easy to find the angle of p2
and then add the angle
calculated above, as shown in this example where the green circle is moved around and the blue position is calculated using the above method:
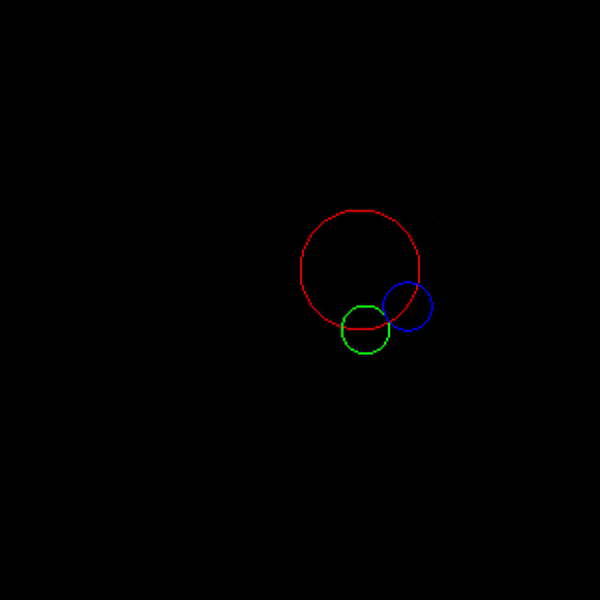
Full source code for a libGDX example:
import com.badlogic.gdx.Game;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.graphics.Color;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.OrthographicCamera;
import com.badlogic.gdx.graphics.glutils.ShapeRenderer;
import com.badlogic.gdx.math.MathUtils;
import com.badlogic.gdx.math.Vector2;
public class SandboxGame extends Game {
OrthographicCamera camera;
ShapeRenderer shapeRenderer;
@Override
public void create () {
float aspectRatio = (float)Gdx.graphics.getHeight()/(float)Gdx.graphics.getWidth();
camera = new OrthographicCamera(10.0f, 10.0f * aspectRatio);
camera.position.set(0, 0, 0);
shapeRenderer = new ShapeRenderer();
}
float b;
@Override
public void render() {
b += 32.0f * Gdx.graphics.getDeltaTime();
Gdx.gl.glClearColor(0, 0, 0, 0);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
camera.update();
shapeRenderer.setProjectionMatrix(camera.combined);
shapeRenderer.begin(ShapeRenderer.ShapeType.Line);
float r = 1.0f;
float rs = 0.4f;
Vector2 p1 = new Vector2(1, 0.5f);
Vector2 p2 = new Vector2(r, 0).rotateDeg(b).add(p1);
float angleToP2 = new Vector2(p2).sub(p1).angleRad();
float angle = 2.0f * MathUtils.asin(rs / r);
Vector2 p3 = new Vector2(r, 0).rotateRad(angleToP2 + angle).add(p1);
shapeRenderer.setColor(Color.RED);
shapeRenderer.circle(p1.x, p1.y, r, 32);
shapeRenderer.setColor(Color.GREEN);
shapeRenderer.circle(p2.x, p2.y, rs, 32);
shapeRenderer.setColor(Color.BLUE);
shapeRenderer.circle(p3.x, p3.y, rs, 32);
shapeRenderer.end();
}
}