This is what my tile map looks like (red highlighting added for clarity):
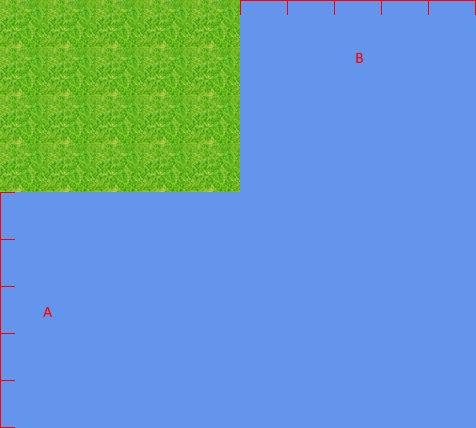
My code:
private List<List<int>> _layoutLayer = new List<List<int>>(50); // Layout Layer
private List<List<int>> _trLayer = new List<List<int>>(50); // Transitional Layer
private List<List<int>> _entityLayer = new List<List<int>>(50); // Object Layer
private List<List<int>> _logicLayer = new List<List<int>>(50); // Logic Layer
// To add a tile
if (inputManager.IsMouseHeld(true))
{
try
{
switch (_currentLayer)
{
case LayerRepresentation.LayoutLayer:
_layoutLayer[_gridMouseY][_gridMouseX] = _currentTile;
break;
case LayerRepresentation.TransitionalLayer:
_trLayer[_gridMouseY][_gridMouseX] = _currentTile;
break;
case LayerRepresentation.EntityLayer:
_entityLayer[_gridMouseY][_gridMouseX] = _currentTile;
break;
case LayerRepresentation.LogicLayer:
_logicLayer[_gridMouseY][_gridMouseX] = _currentTile;
break;
}
}
catch
{
// Player clicked outside of map bounds, add specified amount of tiles to map
}
}
What I need:
When the player clicks outside of map bounds for example on the point A, add the amount of tiles between point A and the map to the map's height. Do the same with point B, but add to the map width instead.
What I've tried:
// Inside the above catch block
if (_mapHeight - _gridMouseY == 0 && _gridMouseX < _mapWidth && _gridMouseX > -1)
{
List<int> tempDefaultLayout = new List<int>();
List<int> tempDefaultOther = new List<int>();
for (int i = 0; i < _layoutLayer[0].Count; i++)
{
tempDefaultLayout.Add(0);
tempDefaultOther.Add(-1);
}
_layoutLayer.Insert(_gridMouseY, tempDefaultLayout);
_trLayer.Insert(_gridMouseY, tempDefaultOther);
_entityLayer.Insert(_gridMouseY, tempDefaultOther);
_logicLayer.Insert(_gridMouseY, tempDefaultOther);
_mapHeight++;
}
else if (_mapWidth - _gridMouseX == 0 && _gridMouseY < _mapHeight && _gridMouseY > -1)
{
for (int y = 0; y < _layoutLayer.Count; y++)
{
for (int x = 0; x < _layoutLayer[y].Count; x++)
{
if (_layoutLayer[y].Count <= _mapWidth)
{
_layoutLayer[y].Add(0);
_trLayer[y].Add(-1);
_entityLayer[y].Add(-1);
_logicLayer[y].Add(-1);
}
}
}
_mapWidth++;
}
return;
The problems:
The first part is about adding tiles to the height (only one row at a time) Adding the temp List
s to the layer List
s with List.Add()
doesn't work, I don't know why. I used List.Insert
instead.
The second part is used to add a single column to the map one at a time.
Loading a map doesn't work because when the loaded map's size is larger than the default programmed size (_mapWidth
and _mapHeight
) in the Map class. My Load()
method:
if (File.Exists(path))
{
float version;
using (BinaryReader reader = new BinaryReader(File.Open(path, FileMode.Open)))
{
version = reader.ReadSingle();
if (version < _version)
{
return -1; // The map you are trying to open has been made with an outdated version of the Editor.
}
if (version > _version)
{
return -2; // The map you are trying to open has been made with a newer version of the Editor.
}
_name = reader.ReadString();
_mapWidth = reader.ReadInt32();
_mapHeight = reader.ReadInt32();
for (int y = 0; y < _mapHeight; y++)
{
for (int x = 0; x < _mapWidth; x++)
{
_layoutLayer[y][x] = reader.ReadInt32();
_trLayer[y][x] = reader.ReadInt32();
_entityLayer[y][x] = reader.ReadInt32();
_logicLayer[y][x] = reader.ReadInt32();
}
}
};
return 0; // Success
}