I am working on a small feature of a 2D mobile game where a indicator points towards a object in 2D space. I am trying to make the indicator turn towards the object however the vector i am trying to construct that points towards the object is off a bit.
When I decided to draw a debug ray from the indicator position to the objects position it was also off a bit, i then decided to draw the debug ray the other way around which gave me a different line. If my understanding is correct, if you switch the starting point and the end point of a ray the line should stay exactly the same right?
using UnityEngine;
using System.Collections.Generic;
public class Tracker : MonoBehaviour {
public GameObject goToTrack;
private List<Renderer> renderers = new List<Renderer>();
void Start(){
foreach (Transform child in transform) {
renderers.Add (child.gameObject.GetComponent<Renderer>());
}
}
void Update () {
}
void OnGUI(){
Vector3 v3Screen = Camera.main.WorldToViewportPoint(goToTrack.transform.position);
// if the target is within the viewable screen the indicator shouldn't be rendered)
if (v3Screen.x > -0.01f && v3Screen.x < 1.01f && v3Screen.y > -0.01f && v3Screen.y < 1.01f) {
foreach (Renderer renderableObject in renderers) {
//renderableObject.enabled = false;
}
}
else
{
foreach (Renderer renderableObject in renderers) {
//renderableObject.enabled = false;
}
v3Screen.x = Mathf.Clamp (v3Screen.x, 0.01f, 0.99f) -0.04f;
v3Screen.y = Mathf.Clamp (v3Screen.y, 0.01f, 0.99f) -0.04f;
transform.position = Camera.main.ViewportToWorldPoint (v3Screen);
Debug.Log ("Target planet position: " + goToTrack.transform.position.ToString());
Debug.DrawRay(Camera.main.ViewportToWorldPoint (v3Screen), goToTrack.transform.position, Color.red, 5.0f);
Debug.DrawRay(goToTrack.transform.position, Camera.main.ViewportToWorldPoint (v3Screen), Color.green, 5.0f);
//Debug.Log ("Tracker angle: " + angle);
//transform.Rotate(0,0, angle, Space.Self);
}
}
}
Output:
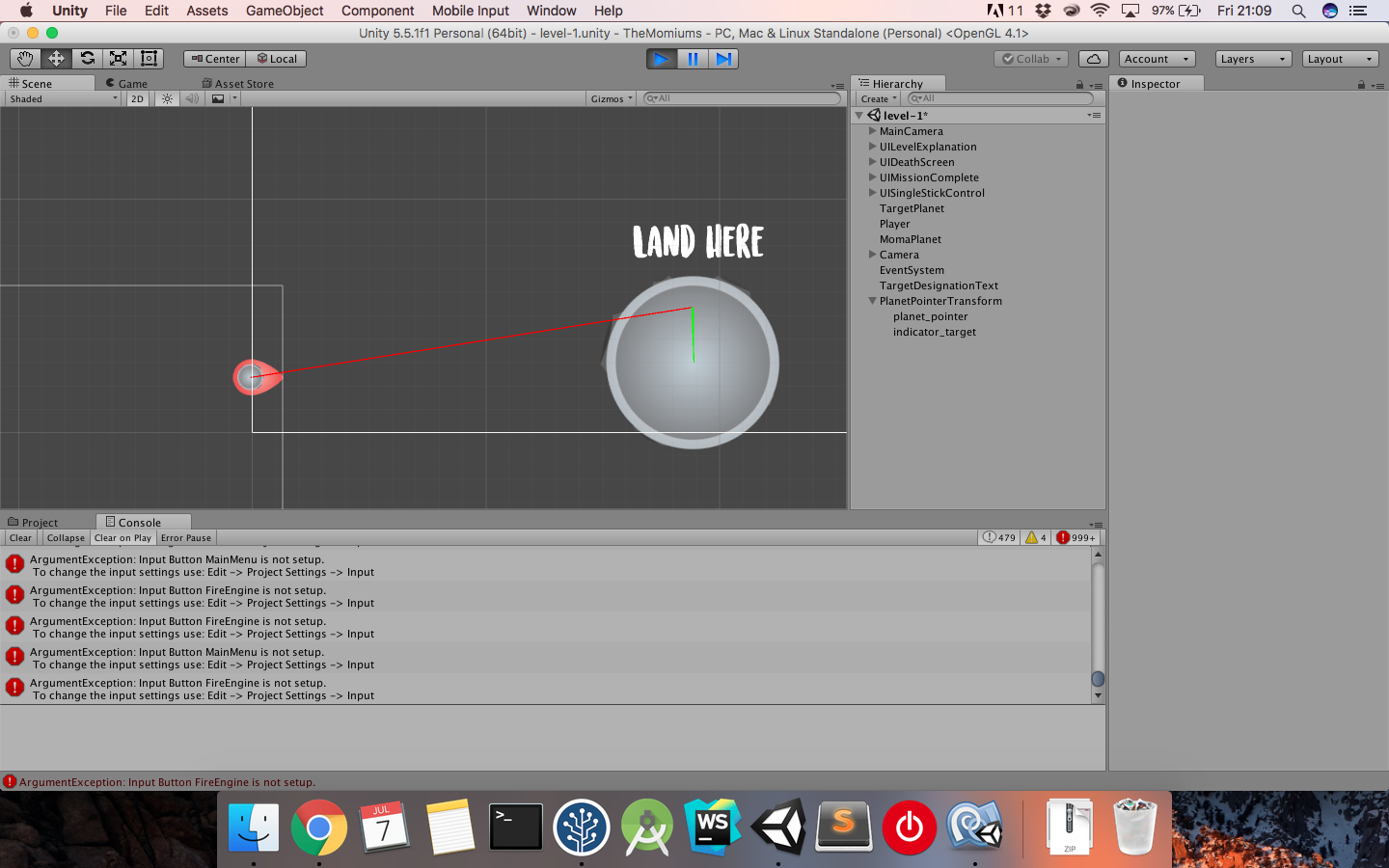